Cross-Browser Automation with Playwright: A Comprehensive Guide
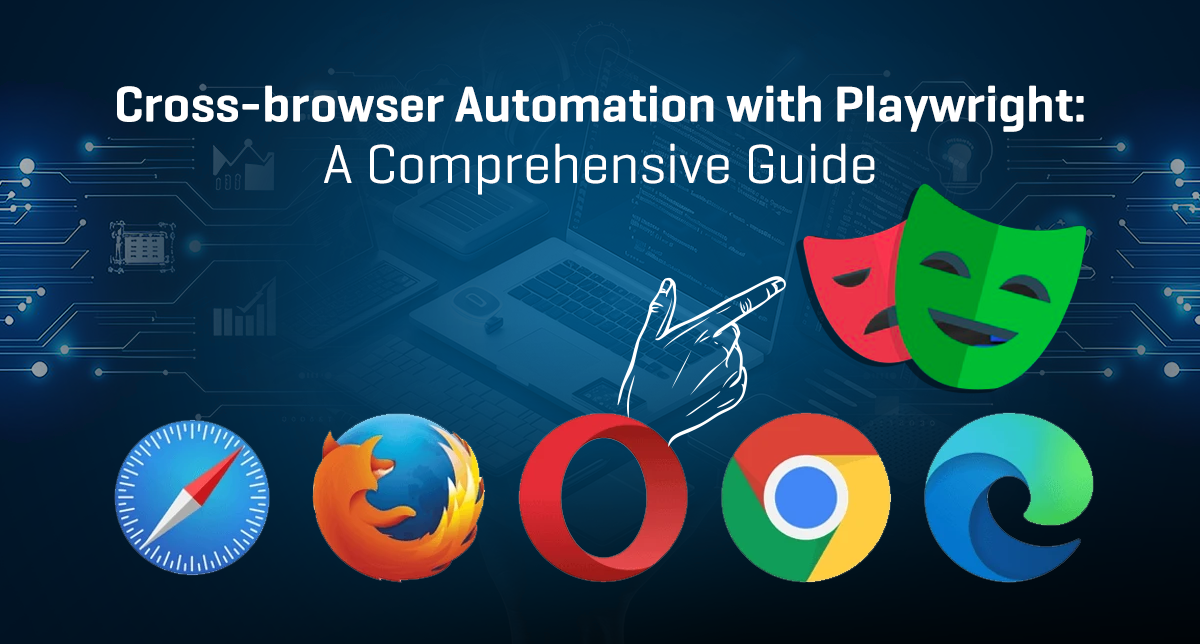
Introduction
The modern web development world is not only focussing on speed but rather compatibility to run across different web browsers seamlessly. Cross-browser automation plays an important factor in providing a consistent, quality user experience on multiple platforms, as a high-quality user experience is seemingly vital to development. This is where Playwright, developed by Microsoft, comes into the picture.
This comprehensive guide is going to dive deeper into the technicalities of using Playwright for high-quality cross-browser automation, including its features, functionalities, and why it is more powerful than existing similar tools.
What is a Playwright?
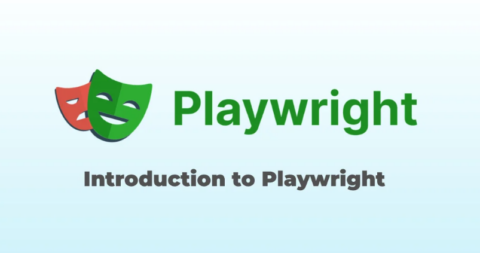
Playwright is an open-source Node.js library. It was developed by Microsoft in 2020 for web browser automation. It offers a single API to drive web content across Chromium, Firefox, and WebKit helping developers to test applications on Chrome, Edge, Safari, and Firefox. This covers 98% of the major browsers we use. With minimal configuration, Playwright gives developers the power to write fast, reliable, and scalable end-to-end tests and supports TypeScript or JavaScript, .NET, and Java.
Key Features of Playwright for Cross-Browser Automation
Single API for Multiple Browsers
Playwright provides a separate, unified API for Chromium, Firefox, and WebKit, meaning a single test suite can be written and those tests can run across these browsers with little to no additional configuration. This refers to less time spent on writing the test suites and maintaining them.
Automatic Waiting and Reliable Execution
Playwright will automatically wait for the flaky test problems to get resolved, the text to be visible, and await all other actionability checks to pass for that element in the end. It will keep on retrying assertions until the expected state is achieved, which means you do not have to write explicit waits.
Browser Contexts and Isolation
Every test runs in an isolated browser context, which prevents tests from interfering with one another. Test context within Browser contexts enables multiple users, sessions, and varying states without affecting other tests.
Support for CI/CD Pipelines
With Docker images, support for headless mode with Playwright makes it a great choice for CI/CD pipelines. It works seamlessly with tools such as GitHub Actions, Jenkins, GitLab, and CircleCI.
Comprehensive Debugging Tools
Playwright has tools like Trace Viewer & Inspector, which give real-time feedback on test interactions, screenshots, and logs that make debugging easy.
Parallel Test Execution and Speed
One of the significant features of Playwright is that it allows concurrent tests by starting multiple browsers and making test execution faster.
Setting Up Playwrightnpm init playwright@latest
Setting up Playwright is less complex and requires minimal configuration. You can start by installing Playwright via npm:
npm init playwright@latest
This command sets up a Playwright project with some default directories/folders for your tests, configuration, and examples.
Example Code for Cross-Browser Testing
The following code snippet shows how to open three browsers (Chromium, Firefox, and WebKit), navigate to a URL, perform a simple action, and log the title of the page:
const { chromium, firefox, webkit } = require('playwright');
(async () => {
const browsers = [chromium, firefox, webkit];
for (const browserType of browsers) {
const browser = await browserType.launch();
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://example.com');
await page.click('button');
await page.waitForSelector('h1');
const title = await page.title();
console.log(`Title on ${browserType.name()}: ${title}`);
await browser.close();
}
})();
Cross-Browser Automation: How Playwright Stands Out
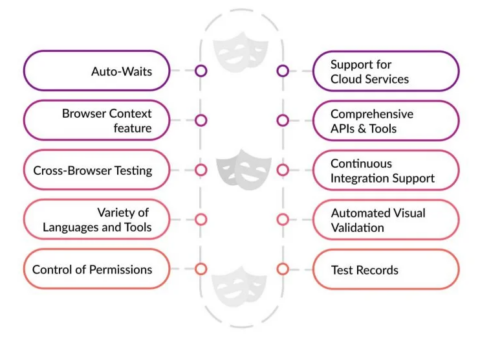
1. Unified Testing for Multiple Browsers
Playwright allows you to automate cross-browser testing on multiple browsers like Chrome, Safari, and Firefox with a single API. While Selenium has separate drivers for each browser, Playwright abstracts these details in its API and similarly treats all browsers.
2. Parallel Testing with Browser Contexts
Browser contexts are essentially isolated, incognito-like browser sessions, which Playwright uses for parallel testing. Contexts run independently of each other and allow for multiple contexts to be run within a single browser instance which can simulate multi-user scenarios.
import { test } from '@playwright/test';
test('test login for User A', async ({ page }) => {
await page.goto('https://example.com');
await page.fill('#username', 'UserA');
await page.fill('#password', 'passwordA');
await page.click('button#submit');
});
test('test login for User B', async ({ page }) => {
await page.goto('https://example.com');
await page.fill('#username', 'UserB');
await page.fill('#password', 'passwordB');
await page.click('button#submit');
});
The tests are executed in their browser contexts, which ensures data from one session doesn’t interfere with another.
3. Mobile Web Testing with Device Emulation
Playwright offers mobile device emulation, which helps developers test how their applications look and perform across various devices and screen sizes.
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const context = await browser.newContext({
viewport: { width: 375, height: 667 },
userAgent: 'Mozilla/5.0 (iPhone; CPU iPhone OS 13_2 like Mac OS X)'
});
const page = await context.newPage();
await page.goto('https://example.com');
await browser.close();
})();
Advanced Features of Playwright
Headless Mode and CI Integration
Playwright supports running with or without a GUI – run tests in headless mode in CI environments. This mode allows quicker execution and consumes less system resources.
To execute tests in headless mode, simply add the headless: true option:
const browser = await chromium.launch({ headless: true });
Network Interception and Mocking
Playwright supports network request interception, which you can use to mock API responses or simulate slow networks.
page.route('**/api/data', route => {
route.fulfill({
status: 200,
contentType: 'application/json',
body: JSON.stringify({ data: 'mocked data' }),
});
});
Tracing and Debugging
Trace Viewer of Playwright is a powerful debugging tool that stores every action that happens in a test run. It provides data for each step of the test with screenshots, network requests, and browser events.
npx playwright show-trace trace.zip
Implementing Playwright with Continuous Integration (CI/CD)
When it comes to integration in your CI/CD pipelines, Playwright offers Docker images and commands for running tests in the headless mode.
Below is an example GitHub Actions configuration for running Playwright tests:
name: Playwright Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Install dependencies
run: npm install
- name: Run Playwright tes
Comparison with Other Testing Frameworks: Selenium, Cypress, and Puppeteer
Feature | Playwright | Selenium | Cypress | Puppeteer |
Languages | JS, TS, Python, .NET, Java | JS, Java, Python, C#, Ruby | JS, TS | JS, TS |
Cross-Browser | Chrome, Firefox, Safari | Chrome, Firefox, Safari, IE | Chrome, Firefox | Chrome, Firefox (limited) |
Headless | Yes | Yes | Yes | Yes |
Mobile Emulation | Yes | No | No | Yes |
Parallel Execution | Yes | No | Limited | Limited |
Best Practices for Playwright
Use Page Objects
Modularize test scripts into page objects, making tests cleaner and easier to maintain.
Enable Automatic Waiting
Playwright waits for an element to appear and be clickable on its own, minimizing flakiness in tests. Do not use explicit waits unless you have to.
Set Up CI Early
If you want to maintain a stable codebase, integrating Playwright tests in the CI pipeline as early as possible is a must to catch the issues early.
Mock Network Requests
For tests that do not rely on real-time data, implement network traffic capture to mock responses. It decreases reliance on external systems and improves test execution speed.
FAQs
1. Does Playwright support cross-browser testing?
Playwright provides cross-browser testing capabilities, allowing developers to automate web applications across the major browsers with a single codebase. This functionality of Playwright enables the testers to write tests once and run them on various browsers without writing separate code from Playwright, which is a great flex for making cross-browser compatibility equal here.
2. Is Playwright good for cross-browser automation?
Since Playwright is robust, quick, and easily maintainable for cross-browser testing automation, it becomes preferable as the best software for cross-browser automation. It supports TypeScript, JavaScript, Python, C#, etc. Playwright relies on page interactions with an auto-waiting mechanism to enhance stability and reduce test flakiness through parallel execution thus making it a great choice for modern automation needs.
3. Which is faster, Selenium or Playwright?
Because of its modern architecture, built-in auto-wait mechanism, and well-handled parallel executions without third-party integrations, Playwright is generally faster than Selenium. Selenium might need more configuration like browser drivers, and it is often hit by flaky tests due to its reduced ability towards DOM tracking.
Playwright has auto-waiting features that make the running of test executions smoother & stable. Additionally, Playwright communicates directly with the browser and offers powerful debugging tools for faster and more reliable automated testing.
Conclusion
Playwright is the preferred cross-browser testing tool offering powerful features and fast execution and reliability. Playwright enables cross-browser testing to be set up with a single API, which fast-tracks the time and complexity involved in getting this up and running through the support of many browsers. It has a built-in debugger, and other tools for parallel execution, mobile device emulation, and network interception, which makes it a powerful software for any test automation framework.
Integrating Playwright with your CI/CD pipeline can provide seamless, dependable test coverage and reduce the time-to-market for web applications. Adopt Playwright to streamline your testing workflows and ensure the delivery of robust web applications is accessible on multiple browsers.