JavaScript Is Both a Blessing and a Curse – Let’s Talk
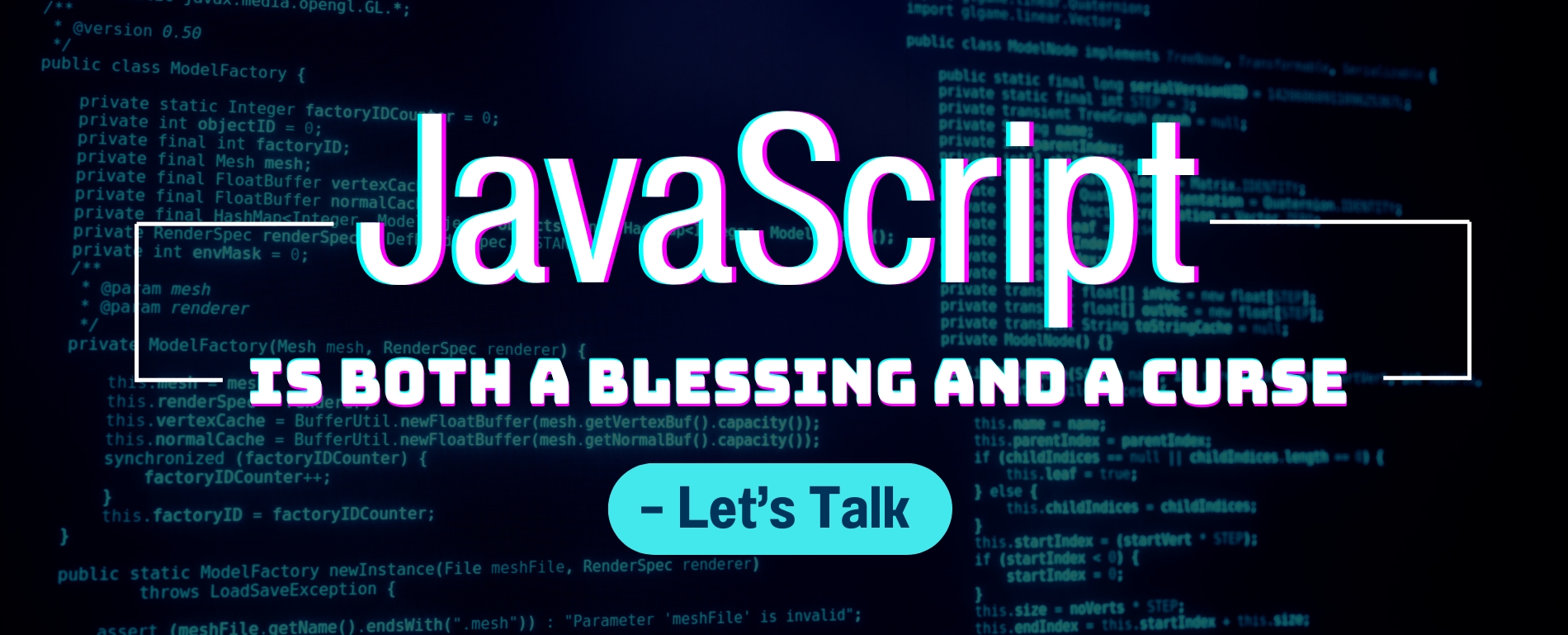
1. Introduction: The Double-Edged Sword of JavaScript
JavaScript is one of the most widely used programming languages in the world. It is the foundation of modern web development and powers almost every interactive website. From simple animations to complex web applications, JavaScript development has shaped how users experience the internet.
A common saying among developers is:
“JavaScript makes everything possible, but not always easy.”
JavaScript frameworks like React, Angular, and Vue have simplified frontend development, while Node.js has expanded its capabilities to the backend. This has made JavaScript a full-stack language, allowing developers to use it across different parts of an application.
Despite its advantages, JavaScript comes with its own set of challenges. Developers often struggle with JavaScript coding challenges such as browser inconsistencies, security vulnerabilities, and maintaining performance in large-scale applications. While the language is flexible, its quirks and unpredictable behavior can sometimes lead to unexpected issues.
This is why JavaScript is both a blessing and a curse. It enables innovation but also presents hurdles that developers must navigate carefully. In this blog, we will explore the pros and cons of JavaScript, discuss real-world case studies, and look at how JavaScript is evolving to meet modern development needs.
2. The Blessing: Why JavaScript Powers the Web
JavaScript is the backbone of modern web applications. It provides the flexibility, speed, and ease of use that developers need to create dynamic and interactive websites. From powering user interfaces to handling backend operations, JavaScript development has revolutionized how websites and applications function. Let’s explore the key advantages that make JavaScript an essential tool for developers.
Ubiquity & Accessibility
One of the biggest strengths of JavaScript development is its ubiquity. JavaScript runs on almost every device and platform. It works seamlessly in browsers, servers, mobile apps, and even IoT devices. This makes it one of the most accessible programming languages.
Because JavaScript is supported by all modern web browsers, developers do not need to install extra software to run JavaScript-based applications. Whether it’s a simple website, a complex web app, or a server-side application using Node.js, JavaScript provides a universal solution for developers.
Additionally, JavaScript enables real-time updates and interactivity. Websites without JavaScript would feel static and unresponsive. Features like dynamic forms, image sliders, live notifications, and chat applications are all powered by JavaScript.
Massive Ecosystem & Community Support
JavaScript has one of the largest and most active developer communities in the world. The npm (Node Package Manager) ecosystem provides access to millions of open-source packages, making development faster and easier.
JavaScript frameworks like React, Angular, Vue, and Svelte help developers build complex applications with reusable components. These frameworks save time and effort by offering pre-built solutions for common development challenges.
Strong community support ensures that developers can quickly find solutions to JavaScript coding challenges. Online resources such as Stack Overflow, GitHub, and MDN Web Docs provide extensive documentation and troubleshooting guides, making it easier for both beginners and experienced developers to improve their JavaScript skills.
Continuous Evolution & Performance Boosts
JavaScript is constantly evolving to meet modern development needs. The V8 engine, which powers Google Chrome and Node.js, continuously improves JavaScript’s execution speed and efficiency. These optimizations make JavaScript applications run faster and consume fewer resources.
New JavaScript frameworks and tools improve both developer experience (DX) and application performance. For example, Svelte compiles JavaScript code into highly optimized, efficient runtime code, reducing browser workload. React’s concurrent rendering and Vue’s reactivity system enhance performance and responsiveness.
Additionally, WebAssembly (Wasm) allows JavaScript to work alongside languages like Rust and C++, unlocking new possibilities for high-performance applications such as gaming, video editing, and AI-powered tools.
Flexibility & Asynchronicity
One of JavaScript’s biggest strengths is its flexibility. Unlike some languages that are restricted to either frontend or backend development, JavaScript can be used for full-stack development. Node.js enables JavaScript to run on servers, allowing developers to build entire applications using a single programming language.
JavaScript also excels in asynchronous programming. With its event-driven, non-blocking I/O model, JavaScript can handle multiple tasks simultaneously without slowing down performance. This is essential for real-time applications like chat apps, live notifications, and online gaming. Technologies such as WebSockets, Promises, and Async/Await help manage asynchronous operations efficiently.
3. The Curse: The Dark Side of JavaScript
Despite its many advantages, JavaScript development comes with its own set of challenges. While it is a powerful and flexible language, its quirks, inconsistencies, and security risks often frustrate developers. From confusing syntax to performance bottlenecks, JavaScript can be difficult to manage without careful coding practices. Let’s explore some of the key issues that make JavaScript both a blessing and a curse.
Inconsistencies & The ‘Weird’ Parts
One of the biggest complaints developers have about JavaScript is its inconsistencies. The language was created quickly in the early days of the web, leading to unexpected behaviors and unusual type conversions.
For example, JavaScript’s type coercion can produce strange results:
console.log([] + []); // "" (empty string)
console.log([] + {}); // "[object Object]"
console.log(1 + "1"); // "11" (string, not a number)
These unpredictable behaviors make debugging difficult and often lead to JavaScript coding challenges that require extra effort to fix.
Additionally, browser inconsistencies create more headaches for developers. Some JavaScript features work differently in Chrome, Firefox, Safari, and Edge, forcing developers to write extra code to ensure cross-browser compatibility.
Framework Fatigue & Over-Reliance on Libraries
The rapid evolution of JavaScript frameworks has made development easier, but it has also created a problem: framework fatigue. New frameworks and tools are introduced frequently, making it difficult for developers to keep up.
For example, React, Angular, Vue, and Svelte are all popular frontend frameworks, but each comes with a unique learning curve. A company might start a project with one framework, only to switch to another later due to performance or scalability issues. This constant shift requires developers to continuously learn and adapt, which can be overwhelming.
Another issue is over-reliance on third-party libraries. The npm ecosystem provides millions of packages, but not all of them are well-maintained. Some projects install hundreds of dependencies, increasing the risk of security vulnerabilities, bloated code, and compatibility issues. A single outdated or compromised package can break an entire application.
Performance & Security Pitfalls
While JavaScript is optimized for performance, poorly written code can slow down applications. Large scripts, excessive DOM manipulations, and inefficient JavaScript coding practices can make websites sluggish.
For example, unoptimized loops, excessive event listeners, and unnecessary re-renders in frameworks like React can degrade user experience. Developers often face challenges in debugging memory leaks and optimizing execution speed.
Security is another major concern in JavaScript development. Because JavaScript runs directly in the browser, it is vulnerable to Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and prototype pollution attacks. If user input is not properly sanitized, attackers can inject malicious scripts that compromise user data.
A notable example is the npm supply chain attack, where hackers injected malicious code into a widely used package, affecting thousands of applications. This highlights the importance of regular security audits and dependency management.
Callback Hell & Async/Await Complexity
JavaScript is designed for asynchronous programming, which is essential for handling multiple tasks at once. However, managing asynchronous operations can be tricky.
In early JavaScript, developers relied on callbacks, but deeply nested callbacks created a mess known as “callback hell”, making code difficult to read and maintain:
doSomething(function(result) {
doSomethingElse(result, function(newResult) {
doAnotherThing(newResult, function(finalResult) {
console.log(finalResult);
});
});
});
To solve this, JavaScript introduced Promises and later Async/Await, which made asynchronous code easier to manage. However, even these solutions have their own JavaScript coding challenges.
For example, poorly structured Async/Await code can lead to race conditions, blocking operations, and unhandled exceptions. Developers need to carefully handle errors and optimize their asynchronous logic to ensure smooth application performance.
4. Case Studies: JavaScript in Real-World Scenarios
Success Story: How JavaScript Transformed Web Apps
JavaScript has helped many companies improve their web applications. One example is Netflix. Initially, Netflix used Java for its backend. Later, it switched to Node.js, a JavaScript runtime. This made the platform faster and improved user experience.
Another success story is React.js. Facebook created React to handle complex user interfaces. Today, it is one of the most popular JavaScript frameworks. Companies like Instagram, Airbnb, and WhatsApp use React for smooth, dynamic web applications.
JavaScript development also powers single-page applications (SPAs). Gmail and Google Docs use JavaScript to update content instantly without reloading the page. This improves performance and user engagement.
Nightmare Story: When JavaScript Went Wrong
JavaScript is powerful, but improper use can cause problems. One example is Twitter’s migration to a heavy JavaScript-based SPA. The website became slow on low-end devices. Later, Twitter had to optimize its JavaScript code to improve speed.
Another example is a security breach in the npm ecosystem. A malicious package was uploaded to npm, affecting thousands of applications. Many projects rely on third-party libraries, making them vulnerable to security risks.
Poorly optimized JavaScript development can also lead to performance issues. Websites with too many scripts load slowly. Users experience delays, which affects business conversions.
These examples show that while JavaScript has many advantages, developers must handle it carefully. Choosing the right JavaScript frameworks, writing clean code, and ensuring security are key to success.
5. The Future: Can JavaScript Fix Its Flaws?
The Rise of Alternative Solutions
JavaScript is powerful, but new technologies are emerging. Deno, created by the same developer behind Node.js, focuses on better security and built-in support for modern features. WebAssembly (Wasm) allows other programming languages to work alongside JavaScript, improving speed and performance.
Another alternative is TypeScript, a superset of JavaScript. TypeScript solves many JavaScript coding challenges by adding static typing, making code more predictable and reducing bugs. Many large companies now prefer TypeScript for safer JavaScript development.
Improvements in JavaScript
JavaScript is constantly improving. The latest ECMAScript (ES) updates introduce better features like optional chaining, nullish coalescing, and top-level await. These improvements make code cleaner and more efficient.
JavaScript frameworks like React, Vue, and Svelte are evolving to improve developer experience. They focus on performance, better state management, and reducing unnecessary re-renders.
The V8 engine continues to optimize JavaScript execution, making applications faster. Features like Just-In-Time (JIT) compilation and memory management are improving.
Should Developers Rethink How They Use JavaScript?
JavaScript is not going away, but developers need to use it wisely. Writing clean, optimized code can prevent performance issues. Choosing the right JavaScript frameworks can reduce complexity. Keeping up with JavaScript development trends helps developers stay ahead.
The future of JavaScript depends on how well it adapts to modern needs. With better tools, improved security, and smarter coding practices, JavaScript can continue to be the foundation of the web.
6. The Business Impact of JavaScript: Why Companies Rely on It
JavaScript is not just a programming language; it is a key driver of digital transformation. Businesses across various industries depend on JavaScript development to create fast, scalable, and user-friendly applications. From e-commerce giants to SaaS companies, JavaScript plays a vital role in delivering seamless digital experiences.
JavaScript’s Role in Digital Transformation
In today’s digital world, businesses need fast, interactive, and scalable applications to stay competitive. JavaScript enables this by powering web applications, mobile apps, and cloud-based platforms. Companies like Amazon, Facebook, Google, and Netflix leverage JavaScript to enhance user experience, optimize performance, and provide dynamic content updates.
- From static websites to interactive apps – JavaScript has transformed how businesses engage with users.
- Cross-platform compatibility – Businesses can use JavaScript across web, mobile, and even IoT devices.
- Full-stack development – With Node.js, JavaScript is used for both frontend and backend development, reducing tech stack complexity.
JavaScript in E-Commerce and SaaS Businesses
JavaScript is a game-changer for e-commerce platforms and SaaS (Software as a Service) applications. These industries require real-time updates, personalized experiences, and high-speed performance—all of which JavaScript delivers.
- E-Commerce:
- JavaScript enables fast-loading product pages, instant cart updates, and personalized recommendations.
- Major platforms like Shopify, Magento, and WooCommerce rely on JavaScript for seamless customer experiences.
- SaaS Applications:
- Tools like Slack, Trello, and Notion use JavaScript to handle real-time collaboration and instant data synchronization.
- JavaScript frameworks like React, Vue, and Angular help build responsive, user-friendly SaaS dashboards.
The Cost of Poor JavaScript Implementation
While JavaScript brings many benefits, poor implementation can negatively impact businesses. A slow or poorly optimized JavaScript application can lead to bad user experience, higher bounce rates, and revenue loss.
- Slow JavaScript performance → Increased bounce rates:
- Studies show that a 1-second delay in page load time can reduce conversions by 7%.
- Heavy JavaScript frameworks and unnecessary dependencies can slow down websites, affecting user engagement.
- Security vulnerabilities → Loss of customer trust:
- Poorly managed JavaScript coding practices can expose businesses to Cross-Site Scripting (XSS) and supply chain attacks.
- Companies need to regularly audit npm dependencies and follow security best practices to protect user data.
- Overuse of third-party libraries → Maintenance issues:
- Relying too much on JavaScript frameworks and libraries can make applications hard to maintain and upgrade.
- Companies need to balance built-in JavaScript solutions with third-party tools to ensure long-term stability.
Conclusion: Embracing JavaScript’s Strengths & Mitigating Its Weaknesses
JavaScript is both a blessing and a curse. It is flexible, widely used, and constantly evolving. It powers modern websites, applications, and backend systems. However, it also has challenges like inconsistencies, security risks, and complex JavaScript development practices.
To make the most of JavaScript, developers should follow best practices. Writing clean and optimized code helps avoid performance issues. Choosing the right JavaScript frameworks can simplify development. Staying updated with modern JavaScript trends ensures better, more efficient coding.
JavaScript is not perfect, but it continues to improve. By understanding its pros and cons, developers can use it effectively and build powerful web applications.
FAQs
1. What are the biggest pros and cons of JavaScript?
Pros: JavaScript is fast, flexible, widely supported, and has a massive ecosystem. It enables interactive web applications and works on both frontend and backend.
Cons: JavaScript has security risks, inconsistent behavior, and can lead to performance issues if not optimized properly.
2. Which JavaScript frameworks are the best for development?
Popular frameworks include React (for UI components), Vue (for simplicity), Angular (for large-scale apps), and Svelte (for performance). The best choice depends on project needs.
3. What are the common JavaScript coding challenges developers face?
Common issues include handling asynchronous code, debugging performance problems, managing dependencies, and fixing browser compatibility errors.
4. Is JavaScript still relevant with new technologies like WebAssembly and Deno?
Yes, JavaScript remains the dominant language for web development. WebAssembly and Deno improve certain aspects but do not replace JavaScript completely. Instead, they work alongside it.
5. How can developers improve JavaScript performance and security?
To improve performance, developers should minimize DOM manipulation, optimize scripts, and use modern JavaScript features. For security, they should validate user input, prevent XSS attacks, and update dependencies regularly.