Optimizing Azure DevOps Pipeline Speed
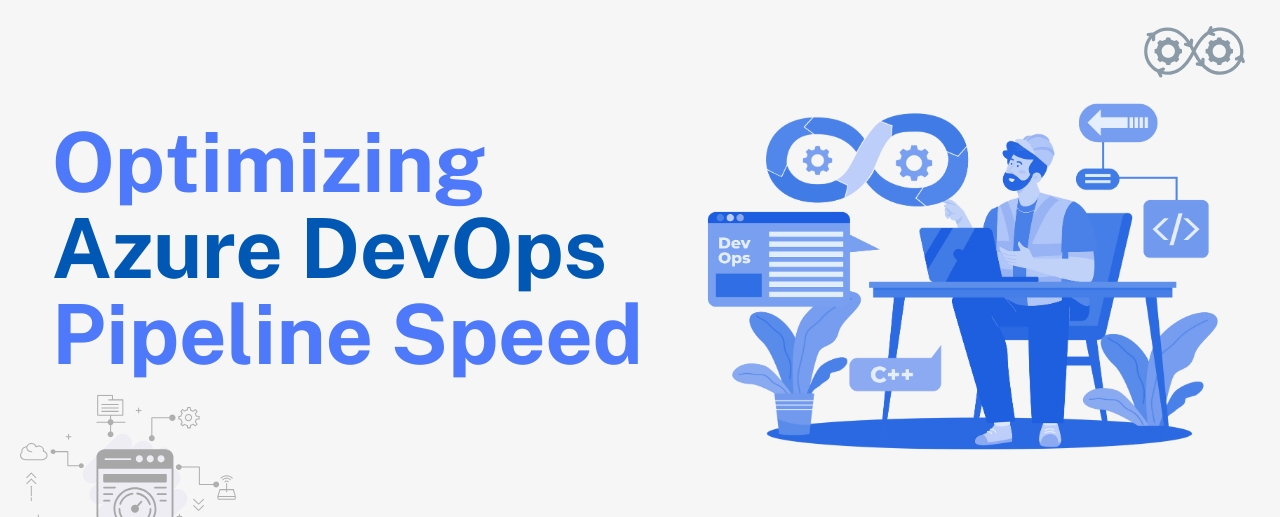
Introduction
As software projects continue to become more complex, no matter if your team is of five or fifty members, the demand for efficient and fast Continuous Integration and Continuous Deployment CI/CD pipelines in Azure DevOps seems to be a greater challenge. A process that starts running smoothly can rapidly come to a grinding halt when the number of features increases, the dependencies grow, and the codebase expands.
A slow pipeline is a headache in big enterprise organizations. When developers have to wait ages for build times or test runs, it delays the probable time for feedback loops, affecting developer productivity. It can directly affect the business agility, by slowing down essential releases, limiting innovation, and upsetting customers.
This is why optimizing the Azure DevOps pipeline for faster and more efficient project delivery is a must. This blog presents some effective strategies to optimize your CI/CD workflows, helping you deliver high-quality applications faster and more reliably using Azure DevOps.
Understanding Pipeline Performance
Before using any of the following optimization strategies, learn which factors influence the pipeline performance. There are multiple stages in the Azure DevOps pipeline; the build, test, and deployment processes. By identifying where the pipeline is slowing down, you can start applying targeted optimizations. The factors slowing down the steps:
- Inefficient Build Tasks: Long-running build tasks, such as compiling code, testing, and packaging.
- Excessive Resource Usage: CPU, RAM, or disk congestion can delay the execution.
- Network Latency: Extracting dependencies from third-party sources may lead to increasing the execution time of the pipeline.
- Complex Dependencies: Handling and installing dependencies, especially in larger projects, may introduce some delays.
Optimizing Build Time
1. Parallelizing Jobs
Parallelizing jobs is one of the most certain ways to make your Azure DevOps pipeline run faster. Instead of running tasks one by one, dividing the jobs into smaller jobs that can run in parallel will lower the total job runtime.
How to Implement:
In your YAML pipeline configuration, you can define multiple jobs and specify that they should run in parallel by using ‘dependsOn’ and ‘condition’. For example:
yaml
jobs:
- job: Build
steps:
- task: BuildTask
- job: Test
dependsOn: [] # This makes it independent and runs in parallel with Build
steps:
- task: TestTask
- job: Publish
dependsOn: [] # This also runs in parallel with Build and Test
steps:
- task: PublishTask
In this case:
- The Build job runs on its own.
- The Test job will run independently, in parallel with Build, because we set dependsOn: [], meaning there are no dependencies.
- The Publish job will also run in parallel with Build and Test for the same reason.
2. Caching Dependencies
The installation of dependencies is yet another common pipeline bottleneck. Whether you are working with npm, .NET, or other package managers, repeatedly downloading the same dependencies can waste significant time.
How to Implement:
Azure DevOps supports caching mechanisms, such as the Cache task, which allows you to cache dependencies between pipeline runs.
Here’s an example of how you can use caching in a ‘Node.js (npm)’ project with Azure DevOps:
yaml
jobs:
- job: Build
steps:
# Cache npm dependencies
- task: Cache@2
inputs:
key: 'npm | "$(Agent.OS)" | package-lock.json'
restoreKeys: |
npm | "$(Agent.OS)"
path: $(Pipeline.Workspace)/.npm
# Install npm dependencies
- script: |
npm install
displayName: 'Install dependencies'
# Run build or other steps
- script: |
npm run build
displayName: 'Build the project'
3. Using Self-Hosted Agents
Although Azure DevOps provides several hosted agents, which are generally more expensive than self-hosted agents, they can be slow because of shared resources. Self-hosted agents are more controlled over env and can be tuned as per your needs.
How to Implement:
Install the Azure Pipelines Agent on your infrastructure if you want to set up a self-hosted agent. Then attach the agent to your Azure DevOps organization. It can help a lot to improve performance, even when you are working on large builds as you can assign more resources to the agent.
Optimizing Deployment Time
1. Smart Deployment Strategies
Deploying to multiple environments sequentially can introduce significant delays. To optimize this, deploy to multiple environments in parallel wherever possible.
How to Implement:
You have the option to define multiple deployment jobs, and they can run in parallel if you configure them in your pipelines. Also, make use of deployment strategies like blue/green or canary releases to reduce downtime.
yaml
jobs:
- job: Build
steps:
- task: Cache@2
inputs:
key: 'npm | "$(Agent.OS)" | package-lock.json'
restoreKeys: |
npm | "$(Agent.OS)"
path: $(Pipeline.Workspace)/.npm
- script: |
npm install
displayName: 'Install dependencies'
- script: |
npm run build
displayName: 'Build the project'
- job: DeployToProduction
dependsOn: Build
condition: succeeded()
steps:
- task: AzureWebApp@1
inputs:
appName: 'MyWebApp'
package: '$(Pipeline.Workspace)/drop/MyWebApp.zip'
displayName: 'Deploy to Production'
- job: DeployToStaging
dependsOn: Build
condition: succeeded()
steps:
- task: AzureWebApp@1
inputs:
appName: 'MyWebApp-Staging'
package: '$(Pipeline.Workspace)/drop/MyWebApp.zip'
displayName: 'Deploy to Staging'
Explanation:
- Parallel Jobs: here you can see two deployment jobs “(DeployToProduction and DeployToStaging)” running in parallel after the build job is completed.
- Deployment Strategies: You can further enhance this by adding deployment strategies like ‘blue/green’ or ‘canary releases’ to ensure smoother rollouts and minimize downtime.
2. Use of Resource Locks
In production deployments, you may come across scenarios where it’s best to lock the environment or the resources to deploy safely. But those locks should only last as long as necessary since holding resources leads to bottlenecks.
How to Implement:
Use resource locking with resource dependencies to prevent your pipeline from waiting when not required. You can leverage Pipeline Conditions as well, to execute jobs only if it makes sense.
Optimizing Pipeline Configuration
1. Minimize Resource Consumption
Consuming too many resources will lead to slowing down your pipeline. For instance, if you need to run a very simple task, running a huge VM (Virtual Machine) is unnecessary.
How to Implement:
Use appropriate VM sizes for your workload. You could use smaller instances for lightweight tasks e.g. “ubuntu-latest” and for more intensive tasks use a more powerful agent to serve resources.
2. Limit Task Execution Scope
Understand what should be run for every pipeline execution. Do not execute unnecessary operations, for example, running heavy tasks (like tests) which are not required.
How to Implement:
Leverage conditional steps or skip conditions to constrain task executions on the outcome of previous tasks and file changes. For example:
YAML
steps:
- task: DotNetCoreCLI@2
condition: and(succeeded(), eq(variables['Build.SourceBranchName'], 'main'))
inputs:
command: 'restore'
projects: '**/*.csproj'
3. Optimize YAML Pipelines
YAML pipelines give you greater flexibility but also come with the responsibility of ensuring they are well-structured. Avoid long-running scripts inside the pipeline itself, which can slow it down.
Few More Best Practices for Optimizing Azure DevOps Pipeline Speed
1. Optimize Artifact Management
Minimize the number of artifacts being passed between pipeline stages to reduce storage and retrieval times.
2. Use YAML Templates
Reuse YAML templates for common tasks across multiple pipelines to simplify configuration and reduce pipeline processing time.
3. Utilize Incremental Builds
Only rebuild parts of the codebase that have changed, instead of rebuilding the entire project.
4. Use Fewer, More Efficient Tasks
Consolidate multiple pipeline tasks into a single, more efficient task to reduce overhead.
5. Prioritize Critical Tasks
Use pipeline priorities or conditions to ensure that critical tasks (like deployment) get faster resources or better priority.
6. Optimize Database Operations
Cache or skip database migrations during non-production builds to save time.
7. Avoid Redundant Builds
Set up logic to ensure that a new build only triggers when necessary (e.g., after a merge or significant change).
FAQs
1. How to optimize a DevOps pipeline?
When it comes to optimizing a DevOps pipeline, here are some of the most strategic options that you can adopt, Parallelize Jobs, Cache Dependencies, and Self Hosted Agent. You can easily eliminate bottlenecks with fewer builds, incremental builds, and quick test execution with parallel tests or test impact analysis. Limit only essential changes to streamline deployments using deployment gates. These are the most popular techniques among many others.
2. What are the benefits of Azure DevOps?
Azure DevOps improves collaboration using built-in version control, automated release and build pipelines, and integration with on-premises and cloud-based resources. Built-in testing helps improve code quality, and it supports CI/CD & tools to help manage projects.
Azure DevOps ensures team productivity, reduces delivery times, and improves alignment between development and operations teams with the help of scalability, flexibility, and advanced security features.
3. How does optimizing a DevOps pipeline benefit a business?
An optimized pipeline for DevOps increases business agility as development cycles are quicker, with fewer manual errors, resulting in higher-quality products. Quicker deployment with higher reliability also means quicker time-to-market allowing a business to adapt to market trends and customer feedback, comparatively faster. This overall contributes to the agility and competitiveness of your business.
Conclusion
While faster builds might be the most obvious benefit of optimizing your Azure DevOps pipeline, the real value lies in freeing up your developer’s time to produce faster and more reliable releases. By adopting unique techniques like parallelization, caching, self-hosted agents, and reduced deployment times, you can reduce the wait time by half or more at a minimal additional effort, and the return on investment will be significant.
Every team is different, so try these tricks and customize your pipeline. What can be the result? Faster releases, more content developers, and a more fluid cycle between planning, development, and the hands-on approach.