10 Expert Performance Tips Every Senior JS React Developer Should Know
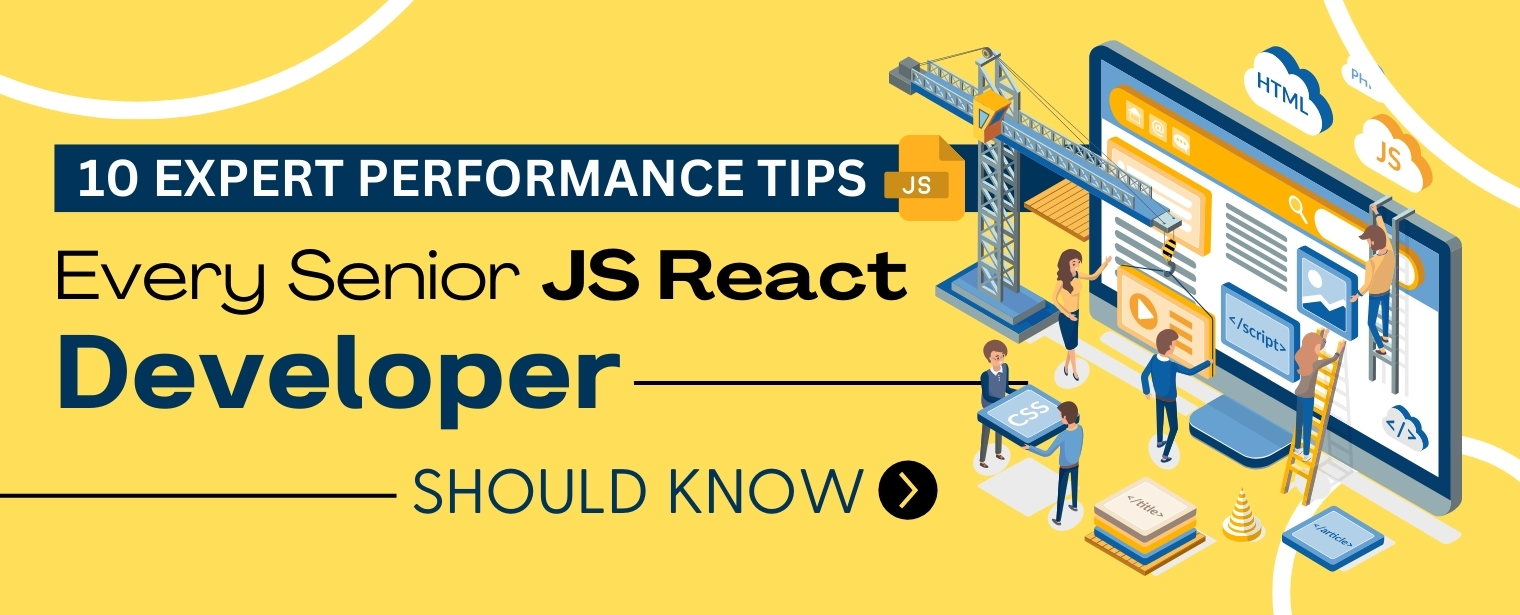
Introduction
As applications grow in complexity, React performance optimization becomes essential for a smooth user experience. Senior developers must go beyond basic techniques to improve efficiency, reduce unnecessary renders, and enhance scalability.
Poorly optimized React applications can lead to slow rendering, high memory usage, and sluggish interactions. Understanding advanced performance strategies can help developers build fast and efficient applications.
In this guide, we will explore 10 expert performance tips that every senior React developer should know. These best practices will help optimize component rendering, improve state management, and enhance overall application speed.
1. Optimize Rendering with React Memoization Techniques
React re-renders components whenever their state or props change. While this ensures UI updates, unnecessary re-renders can slow down performance. Memoization helps optimize rendering by preventing components from re-rendering when their data has not changed.
Using React.memo() to Prevent Unnecessary Re-renders
React.memo() is a higher-order component that memorizes the output of a functional component. If the props remain the same, React reuses the previous result instead of re-rendering the component.
Example:
const MemoizedComponent = React.memo(({ data }) => {
console.log("Rendered");
return <div>{data}</div>;
});
This ensures the component only re-renders when data changes.
Optimizing Functional Components with useMemo() and useCallback()
- useMemo() caches computed values to avoid recalculating them on every render.
- useCallback() memoizes functions so that they do not get re-created unnecessarily.
Example:
const computedValue = useMemo(() => expensiveCalculation(value), [value]);
const handleClick = useCallback(() => console.log("Clicked"), []);
This improves performance by reducing unnecessary calculations and function re-creation.
Avoiding Prop Drilling That Causes Unnecessary Re-renders
Passing props deeply through multiple components can lead to wasted renders. Instead, use:
- Context API to manage shared state globally.
- useReducer for structured state updates.
By reducing prop drilling, components update only when necessary.
Example: Reducing Re-renders in a List Component with React.memo()
const ListItem = React.memo(({ item }) => {
console.log("Item rendered:", item.id);
return <li>{item.name}</li>;
});
const List = ({ items }) => {
return (
<ul>
{items.map((item) => (
<ListItem key={item.id} item={item} />
))}
</ul>
);
};
Here, ListItem only re-renders when its specific item changes, not when the parent List re-renders.
By using React.memo(), useMemo(), and useCallback(), developers can prevent unnecessary renders, reduce memory usage, and improve overall React application performance.
2. Implement Server-Side Rendering (SSR) and Static Site Generation (SSG)
Rendering strategy plays a crucial role in React performance and SEO. By default, React applications use Client-Side Rendering (CSR), where the browser loads JavaScript, fetches data, and renders content dynamically. However, this can cause slow initial loading and poor SEO. Server-Side Rendering (SSR) and Static Site Generation (SSG) help optimize performance and improve user experience.
Why SSR and SSG Matter for Performance and SEO
- Faster initial load time – Pages are pre-generated instead of being built dynamically in the browser.
- Better SEO – Search engines can easily crawl and index fully rendered pages.
- Improved user experience – Users see content faster instead of waiting for client-side JavaScript execution.
Using Next.js for SSR: Hydrating React Components on the Server
Server-Side Rendering (SSR) generates the HTML for a page on the server before sending it to the browser. This reduces the time required for the first paint. Next.js makes SSR implementation straightforward.
Example: Implementing SSR in Next.js
export async function getServerSideProps() {
const data = await fetchDataFromAPI();
return { props: { data } };
}
const Page = ({ data }) => {
return <div>{data.title}</div>;
};
export default Page;
- The getServerSideProps function fetches data on each request and sends the fully rendered HTML to the client.
- This improves Time to First Byte (TTFB) and makes the page immediately visible to users.
Using SSG for Static Content: Pre-Rendering at Build Time
For pages that do not change often, Static Site Generation (SSG) is the best approach. The page is pre-built during deployment, making it load instantly.
Example: Implementing SSG in Next.js
export async function getStaticProps() {
const data = await fetchDataFromAPI();
return { props: { data } };
}
const Page = ({ data }) => {
return <div>{data.title}</div>;
};
export default Page;
- The getStaticProps function fetches data at build time and pre-renders the page.
- This reduces server load and enables ultra-fast loading for users.
Best Practices for Choosing Between SSR, SSG, and CSR
Rendering Strategy | Best For | Considerations |
SSR (Server-Side Rendering) | Dynamic data, SEO, fast initial render | Can increase server load |
SSG (Static Site Generation) | Blogs, documentation, marketing pages | Not suitable for frequently changing data |
CSR (Client-Side Rendering) | Single-page apps, dashboards | Slower initial load, requires hydration |
- Use SSR when data is frequently updated and SEO is critical.
- Use SSG when content is mostly static and needs fast loading.
- Use CSR for interactive apps where SEO is not a priority.
By choosing the right rendering strategy, developers can improve performance, enhance user experience, and optimize SEO in React applications.
3. Utilize Immutable Data Structures for Efficient State Management
Efficient state management is essential for maintaining React performance. One of the best practices to achieve this is by using immutable data structures. Immutable state updates ensure that changes do not modify the original data but instead create a new copy. This helps prevent unnecessary re-renders and improves React app performance.
How Immutable Data Structures Prevent Unnecessary Re-Renders
React determines whether a component should re-render by comparing previous state with current state. If the reference remains the same, React skips rendering. However, when mutable state updates are used, React cannot detect changes efficiently, leading to unnecessary re-renders.
With immutable data structures, a new object is created whenever the state is updated, allowing React’s reconciliation process to work optimally.
Using Libraries Like Immutable.js or Immer for Managing Immutable State
Manually handling immutable updates in JavaScript can be complex. Libraries like Immutable.js and Immer make it easier by providing structured, immutable data manipulation.
Using Immutable.js:
import { Map } from "immutable";
const initialState = Map({ count: 0 });
// Creating a new immutable state instead of modifying the original
const newState = initialState.set("count", initialState.get("count") + 1);
console.log(initialState === newState); // false (immutable update)
- The .set() method returns a new state object instead of modifying the existing one.
- This ensures efficient change detection, reducing unnecessary renders.
Using Immer:
import produce from "immer";
const initialState = { count: 0 };
const newState = produce(initialState, (draft) => {
draft.count += 1;
});
console.log(initialState === newState); // false (immutable update)
- Immer simplifies immutable updates by allowing mutations inside a draft function.
- It creates a new state object while maintaining immutability.
The Role of Redux and React Context API in Managing State Efficiently
For larger applications, Redux and the React Context API help manage global state efficiently. Both follow the principle of immutable state updates.
Using Redux with Immutability:
const reducer = (state = { count: 0 }, action) => {
switch (action.type) {
case "INCREMENT":
return { ...state, count: state.count + 1 }; // Immutable update
default:
return state;
}
};
- The spread operator (…state) ensures a new state object is returned.
- This prevents direct state mutations, improving Redux performance.
Using React Context API with Immutable State:
const initialState = { count: 0 };
const CountContext = createContext(initialState);
const CountProvider = ({ children }) => {
const [state, setState] = useState(initialState);
const increment = () => {
setState((prev) => ({ ...prev, count: prev.count + 1 })); // Immutable update
};
return (
<CountContext.Provider value={{ state, increment }}>
{children}
</CountContext.Provider>
);
};
- Using the spread operator ensures that previous state is not modified directly.
- React Context API works best when combined with immutable state management.
Example: How Immutable State Updates Improve React Component Performance
Consider a scenario where a list component re-renders unnecessarily due to mutable state updates.
Without Immutable State:
const [items, setItems] = useState(["Apple", "Banana"]);
const addItem = (item) => {
items.push(item); // Mutable update
setItems(items); // React cannot detect change efficiently
};
- Since push() modifies the original array, React does not detect changes, causing performance issues.
With Immutable State:
const [items, setItems] = useState(["Apple", "Banana"]);
const addItem = (item) => {
setItems((prevItems) => [...prevItems, item]); // Immutable update
};
- The spread operator creates a new array, making it easier for React to detect changes and optimize re-renders.
4. Optimize Asset Loading with Code Splitting and Lazy Loading
Efficient asset loading is crucial for improving React application performance. Large JavaScript bundles slow down the initial page load, especially on low-bandwidth networks. Code splitting and lazy loading help reduce the initial bundle size, ensuring faster load times and a smoother user experience.
Reducing Initial Bundle Size with Code Splitting
By default, React applications bundle all JavaScript code into a single file. This can lead to slow page loads and higher memory usage. Code splitting divides the bundle into smaller chunks, loading only what is needed. This significantly improves load time and performance.
Using Dynamic Imports to Split Code at Logical Points
Dynamic imports allow developers to load specific modules only when required, reducing initial load time. Webpack’s import() function makes it easy to split code dynamically.
Example: Code Splitting with Dynamic Imports
import("./heavyComponent").then((module) => {
const HeavyComponent = module.default;
// Use HeavyComponent when needed
});
- This ensures that HeavyComponent is not included in the initial bundle.
- The browser downloads it only when needed, reducing page load time.
React.lazy() and Suspense for Lazy-Loading Non-Critical Components
React.lazy() simplifies lazy loading by dynamically loading components. It works best with React Suspense, which provides a fallback while the component loads.
Example: Lazy Loading a Component with React.lazy() and Suspense
import React, { Suspense, lazy } from "react";
// Lazy load the component
const HeavyComponent = lazy(() => import("./HeavyComponent"));
const App = () => {
return (
<div>
<h1>React Performance Optimization</h1>
<Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</Suspense>
</div>
);
};
export default App;
- React.lazy() loads HeavyComponent only when needed, keeping the initial bundle lightweight.
- Suspense ensures a smooth user experience by displaying a fallback UI while loading.
Example: Deferring Component Rendering with React.lazy() and Suspense
Lazy loading is beneficial for non-essential UI elements such as charts, modals, or third-party widgets.
Without Lazy Loading (Inefficient Approach)
import HeavyChart from "./HeavyChart";
const Dashboard = () => {
return (
<div>
<h1>Dashboard</h1>
<HeavyChart />
</div>
);
};
- The HeavyChart component loads immediately, even if the user does not interact with it.
With Lazy Loading (Optimized Approach)
const HeavyChart = lazy(() => import("./HeavyChart"));
const Dashboard = () => {
return (
<div>
<h1>Dashboard</h1>
<Suspense fallback={<div>Loading chart...</div>}>
<HeavyChart />
</Suspense>
</div>
);
};
- The HeavyChart component loads only when required, reducing initial load time.
By implementing code splitting and lazy loading, React applications become faster and more responsive, ensuring a better user experience.
5. Employ Progressive Web App (PWA) Techniques for Faster Performance
PWAs improve React application performance by enhancing speed, reliability, and offline capabilities. They leverage service workers and caching strategies to optimize load times.
Implementing Service Workers for Caching
Service workers store static assets and API responses, reducing server requests.
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
Using a Web App Manifest for an App-Like Experience
The manifest.json defines how the PWA behaves and appears.
{
"short_name": "ReactPWA",
"name": "React Progressive Web App",
"start_url": "/",
"display": "standalone"
}
Example: Improving Offline Functionality with Service Workers
If a user is offline, cached responses ensure uninterrupted browsing.
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).catch(() => caches.match('/offline.html'))
);
});
By caching key resources and supporting offline access, PWAs enhance performance, user engagement, and reliability in React applications.
6. Minimize and Optimize CSS and JavaScript Delivery
Optimizing CSS and JavaScript delivery is essential for improving React application performance. Large and unoptimized assets can slow down page load times, affect SEO rankings, and degrade user experience. By minimizing, compressing, and strategically loading CSS and JavaScript files, applications can achieve faster rendering and better efficiency.
Why Reducing CSS and JS File Sizes Improves Performance
Browsers must download, parse, and execute CSS and JavaScript files before rendering a webpage. Large files lead to:
- Slow initial page loads, especially on mobile networks.
- Increased memory usage, impacting performance on low-end devices.
- Render-blocking issues, delaying the display of visible content.
By optimizing asset delivery, React applications can load faster and provide a smoother user experience.
Minifying Assets with Tools Like UglifyJS and CSSNano
Minification removes unnecessary characters (like whitespace, comments, and redundant code) from CSS and JavaScript files, reducing their size.
- UglifyJS: A popular tool for minifying JavaScript.
- CSSNano: A powerful CSS minifier that removes unused styles and optimizes CSS delivery.
- Terser: A modern JavaScript minifier used in Webpack for reducing bundle sizes.
Example: Minifying JavaScript with Terser in Webpack
const TerserPlugin = require("terser-webpack-plugin");
module.exports = {
optimization: {
minimize: true,
minimizer: [new TerserPlugin()],
},
};
- This configuration ensures JS files are compressed for production.
Example: Minifying CSS with CSSNano in Webpack
const CssMinimizerPlugin = require("css-minimizer-webpack-plugin");
module.exports = {
optimization: {
minimize: true,
minimizer: [new CssMinimizerPlugin()],
},
};
- This setup removes redundant styles and compresses CSS files.
Inlining Critical CSS to Load Essential Styles Faster
Instead of loading an entire CSS file upfront, critical CSS can be inlined directly within the HTML. This improves the First Contentful Paint (FCP) by displaying essential styles immediately.
Example: Inlining Critical CSS in React
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
</style>
- This allows important styles to load without waiting for an external CSS file.
For larger projects, tools like CriticalCSS can automate this process by extracting above-the-fold styles and inlining them.
Example: Using Webpack to Minimize JS and CSS for Production
A production-ready Webpack configuration ensures CSS and JS are optimized for performance.
const MiniCssExtractPlugin = require("mini-css-extract-plugin");
const CssMinimizerPlugin = require("css-minimizer-webpack-plugin");
const TerserPlugin = require("terser-webpack-plugin");
module.exports = {
mode: "production",
module: {
rules: [
{
test: /\.css$/,
use: [MiniCssExtractPlugin.loader, "css-loader"],
},
],
},
optimization: {
minimize: true,
minimizer: [new TerserPlugin(), new CssMinimizerPlugin()],
},
plugins: [new MiniCssExtractPlugin()],
};
- MiniCssExtractPlugin extracts and minimizes CSS for faster page loads.
- TerserPlugin ensures JavaScript files are compressed.
By minimizing and optimizing CSS and JavaScript delivery, React applications can load faster, use fewer resources, and provide a better user experience.
7. Reduce Reconciliation Workload with Efficient DOM Updates
Efficient DOM updates are critical for improving React application performance. React uses a virtual DOM to update only the necessary parts of the UI. However, inefficient updates can increase the reconciliation workload, leading to slow rendering and unnecessary re-renders. By understanding React’s reconciliation process and using best practices like key props and efficient list rendering, developers can enhance performance and responsiveness.
Understanding React’s Reconciliation Process
React’s reconciliation algorithm determines how the UI should update when the state changes. It follows these key steps:
- Compares the virtual DOM with the previous render to detect changes.
- Updates only the modified elements instead of re-rendering the entire DOM.
- Uses diffing and tree comparison to determine the most efficient way to update the UI.
However, inefficient component updates can cause unnecessary re-rendering, leading to performance bottlenecks.
Using Key Props Effectively to Optimize List Rendering
When rendering lists, React relies on key props to track individual items. Without proper keys, React re-renders entire lists, reducing performance.
Bad Practice: Using Index as Key
const List = ({ items }) => {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item.name}</li> // Avoid using index as a key
))}
</ul>
);
};
- This can cause incorrect reordering when items are added or removed.
Best Practice: Using Unique Identifiers as Key
const List = ({ items }) => {
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li> // Use a unique ID as the key
))}
</ul>
);
};
- This ensures efficient updates without affecting unchanged list items.
Avoiding Deep Object Mutations That Cause Unnecessary Re-Renders
React’s reconciliation detects changes by shallow comparison. If a deeply nested object is mutated, React assumes the entire object has changed, triggering unnecessary re-renders.
Bad Practice: Mutating State Directly
const handleUpdate = () => {
user.profile.age = 30; // Mutating object directly
setUser(user); // React does not detect the change properly
};
- This does not trigger a re-render because the reference to user remains unchanged.
Best Practice: Using Immutable Updates
const handleUpdate = () => {
setUser((prevUser) => ({
...prevUser,
profile: { ...prevUser.profile, age: 30 }, // Creates a new object reference
}));
};
- This ensures React detects the update and re-renders only the necessary components.
Example: Optimizing Large Lists with react-window
When rendering large lists, React can struggle with slow performance due to excessive DOM updates. The react-window library helps by virtualizing lists, rendering only visible items.
Without Virtualization (Inefficient for Large Lists)
const LargeList = ({ items }) => {
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
};
- React renders all list items, even those not visible on the screen.
With react-window (Optimized for Performance)
import { FixedSizeList as List } from "react-window";
const LargeList = ({ items }) => {
return (
<List height={400} width={300} itemSize={50} itemCount={items.length}>
{({ index, style }) => (
<div style={style}>{items[index].name}</div>
)}
</List>
);
};
- Only visible items are rendered, reducing the reconciliation workload and improving performance.
By optimizing DOM updates, using efficient key props, preventing deep object mutations, and implementing list virtualization, React applications can achieve faster rendering, reduced re-renders, and better overall performance.
8. Implement Virtualization for Large Lists and Grids
Rendering large lists and grids in React applications can severely impact performance. When a list contains thousands of items, React renders all elements in the DOM, even those not visible on the screen. This increases memory usage, slows down UI interactions, and affects rendering speed.
Virtualization solves this problem by rendering only the visible items in the viewport while dynamically loading additional elements as the user scrolls. Libraries like react-window and react-virtualized optimize list and grid rendering, improving performance and UI responsiveness.
Why Rendering Large Lists Affects React Performance
When dealing with long lists or complex grids, React follows these steps:
- Creates and maintains all list items in the virtual DOM.
- Re-renders all elements whenever state or props change.
- Increases memory consumption and slows down UI responsiveness.
The more items React processes, the slower the application becomes. This issue becomes worse on lower-end devices with limited resources.
Problem Example: Rendering a Large List Without Optimization
const LargeList = ({ items }) => {
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li> // Rendering all items at once
))}
</ul>
);
};
- Problem: React loads and renders every item, even those outside the viewport.
Using react-window and react-virtualized for Efficient Rendering
To optimize list and grid rendering, we can use react-window or react-virtualized, which only render visible components while efficiently handling scrolling.
Optimized Example: Using react-window for List Virtualization
import { FixedSizeList as List } from "react-window";
const VirtualizedList = ({ items }) => {
return (
<List height={400} width={300} itemSize={50} itemCount={items.length}>
{({ index, style }) => (
<div style={style}>{items[index].name}</div>
)}
</List>
);
};
- Only the visible items are rendered, reducing DOM load and improving performance.
- Smooth scrolling without performance lag.
Optimized Example: Using react-virtualized for Grid Virtualization
import { Grid } from "react-virtualized";
const VirtualizedGrid = ({ items }) => {
return (
<Grid
cellRenderer={({ columnIndex, key, rowIndex, style }) => (
<div key={key} style={style}>
{items[rowIndex][columnIndex]}
</div>
)}
columnCount={3}
columnWidth={100}
height={400}
rowCount={items.length / 3}
rowHeight={50}
width={300}
/>
);
};
- Only the visible grid cells are rendered, avoiding unnecessary re-renders.
- Improves UI responsiveness, especially for large datasets.
Example: Virtualizing a Large Dataset to Improve UI Responsiveness
Before Virtualization (Slow Performance)
- Rendering 10,000 list items at once.
- High memory usage.
- Slow scrolling and delayed UI updates.
After Virtualization (Optimized Performance)
- Only renders visible elements (~10-20 items at a time).
- Reduces memory usage significantly.
- Smooth scrolling with faster UI updates.
9. Optimize API Calls and Asynchronous Data Fetching
Efficient API handling is crucial for React performance. Poorly optimized API requests can lead to slow page loads, excessive re-renders, and unnecessary network requests, affecting user experience. By implementing pagination, caching, and debouncing techniques, developers can enhance performance and reduce server load.
Reducing API Over-Fetching with Proper Pagination and Caching
One of the common performance issues in React applications is fetching large amounts of data at once. Instead of loading everything at once, implementing pagination, infinite scrolling, or caching ensures that only the necessary data is retrieved when needed.
Example: Fetching Paginated Data to Reduce Load
const fetchUsers = async (page) => {
const response = await fetch(`https://api.example.com/users?page=${page}`);
return response.json();
};
const PaginatedUsers = ({ page }) => {
const [users, setUsers] = useState([]);
useEffect(() => {
fetchUsers(page).then((data) => setUsers(data));
}, [page]);
return (
<ul>
{users.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
};
- Only loads a subset of data per request, reducing API load.
- Prevents over-fetching and improves response times.
Using React Query, SWR, or Apollo Client for Efficient Data Fetching
Instead of manually handling API calls, React Query, SWR, or Apollo Client can simplify data fetching, caching, and background updates.
Example: Implementing Caching with React Query
import { useQuery } from "react-query";
const fetchUsers = async () => {
const response = await fetch("https://api.example.com/users");
return response.json();
};
const UsersList = () => {
const { data, error, isLoading } = useQuery("users", fetchUsers);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error loading users</p>;
return (
<ul>
{data.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
};
- Built-in caching prevents redundant API requests.
- Automatically re-fetches and updates stale data in the background.
- Improves performance by reducing unnecessary re-renders.
Debouncing and Throttling API Requests to Prevent Excessive Rendering
When handling search inputs, live filtering, or real-time updates, excessive API calls can slow down performance. Debouncing and throttling help in reducing frequent requests, ensuring that the API is not overwhelmed.
Example: Debouncing API Calls in React
import { useState, useEffect } from "react";
const fetchResults = async (query) => {
const response = await fetch(`https://api.example.com/search?q=${query}`);
return response.json();
};
const SearchComponent = () => {
const [query, setQuery] = useState("");
const [results, setResults] = useState([]);
useEffect(() => {
const timer = setTimeout(() => {
if (query) {
fetchResults(query).then((data) => setResults(data));
}
}, 500); // Debounce time: 500ms
return () => clearTimeout(timer);
}, [query]);
return (
<div>
<input
type="text"
placeholder="Search..."
onChange={(e) => setQuery(e.target.value)}
/>
<ul>
{results.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
- Delays API requests until the user stops typing, reducing unnecessary calls.
- Prevents lag and excessive rendering during rapid input changes.
10. Enhance Performance with Worker Threads and WebAssembly
As React applications grow in complexity, handling heavy computations on the main thread can slow down rendering and impact user experience. Worker threads and WebAssembly (WASM) help offload tasks to separate execution environments, improving performance and responsiveness.
How Web Workers Offload Heavy Computations to Background Threads
The main thread in a browser is responsible for handling UI updates and user interactions. When it processes CPU-intensive tasks, the UI may become sluggish or unresponsive. Web Workers allow developers to run scripts in background threads, ensuring the main thread remains free for rendering and interactivity.
Example: Running Complex Calculations with Web Workers
Create a separate worker script (worker.js):
self.onmessage = function (event) {
let result = 0;
for (let i = 0; i < event.data; i++) {
result += i;
}
self.postMessage(result);
};
Use the worker in a React component:
import { useState, useEffect } from "react";
const HeavyComputation = () => {
const [result, setResult] = useState(null);
useEffect(() => {
const worker = new Worker(new URL("./worker.js", import.meta.url));
worker.postMessage(100000000); // Send computation task
worker.onmessage = (event) => {
setResult(event.data);
worker.terminate();
};
}, []);
return <p>Computation Result: {result}</p>;
};
- Moves intensive calculations to a worker thread to keep the UI smooth.
- Reduces blocking on the main thread, improving React’s responsiveness.
Using WebAssembly to Boost React Performance for Computation-Heavy Tasks
WebAssembly (WASM) is a low-level binary format that enables near-native performance in web applications. React applications that require image processing, cryptography, or physics simulations can leverage WebAssembly for optimized performance.
Steps to Integrate WebAssembly in React
- Write high-performance code in C/C++ or Rust.
- Compile the code into a WebAssembly module.
- Load and use the compiled WASM module in React.
Example: Running a WebAssembly Module in React
- Compile a simple Rust function into WebAssembly:
#[no_mangle]
pub extern "C" fn add_numbers(a: i32, b: i32) -> i32 {
a + b
}
- Import the WebAssembly module in React:
import { useState, useEffect } from "react";
const loadWASM = async () => {
const wasm = await WebAssembly.instantiateStreaming(
fetch("module.wasm")
);
return wasm.instance.exports;
};
const WebAssemblyDemo = () => {
const [sum, setSum] = useState(null);
useEffect(() => {
loadWASM().then((wasm) => {
setSum(wasm.add_numbers(10, 20)); // Call WASM function
});
}, []);
return <p>Sum from WebAssembly: {sum}</p>;
};
- Processes complex calculations much faster than JavaScript alone.
- Optimizes computationally expensive operations, enhancing React app performance.
By leveraging Web Workers and WebAssembly, React developers can significantly improve performance for CPU-intensive applications, ensuring a smooth and efficient user experience.
Conclusion
Optimizing React performance is essential for building scalable, efficient, and responsive applications. By implementing best practices like memoization, server-side rendering, state management optimization, and virtualization, developers can significantly enhance rendering efficiency. Additionally, leveraging Web Workers and WebAssembly ensures that heavy computations do not block the main thread, maintaining a smooth user experience.
As React applications continue to grow in complexity, staying updated with modern optimization techniques is key to maintaining high-performance standards. By following these expert performance tips, senior developers can build React applications that are not only fast but also future-ready.
FAQs
1. Why is memoization important for React performance?
Memoization prevents unnecessary re-renders by caching the results of expensive calculations or component outputs. Using React.memo(), useMemo(), and useCallback() helps optimize rendering and improve efficiency.
2. How does SSR improve React app performance?
Server-Side Rendering (SSR) improves first-page load time and SEO by pre-rendering content on the server. It ensures that users receive a fully-rendered page quickly, enhancing performance and search engine visibility.
3. What are the benefits of using virtualization in React?
Virtualization tools like react-window and react-virtualized reduce memory usage and improve rendering speed by only rendering the visible portion of a large list or grid. This prevents performance issues in data-heavy applications.
4. How does WebAssembly improve performance in React apps?
WebAssembly (WASM) executes CPU-intensive tasks at near-native speeds, significantly improving performance for tasks like image processing, cryptography, and physics simulations in React applications.
5. What are the best tools for optimizing API calls in React?
Libraries like React Query, SWR, and Apollo Client manage API caching, pagination, and request deduplication, reducing over-fetching and improving the efficiency of asynchronous data fetching.