API development: Ultimate Guide to Developing Robust APIs
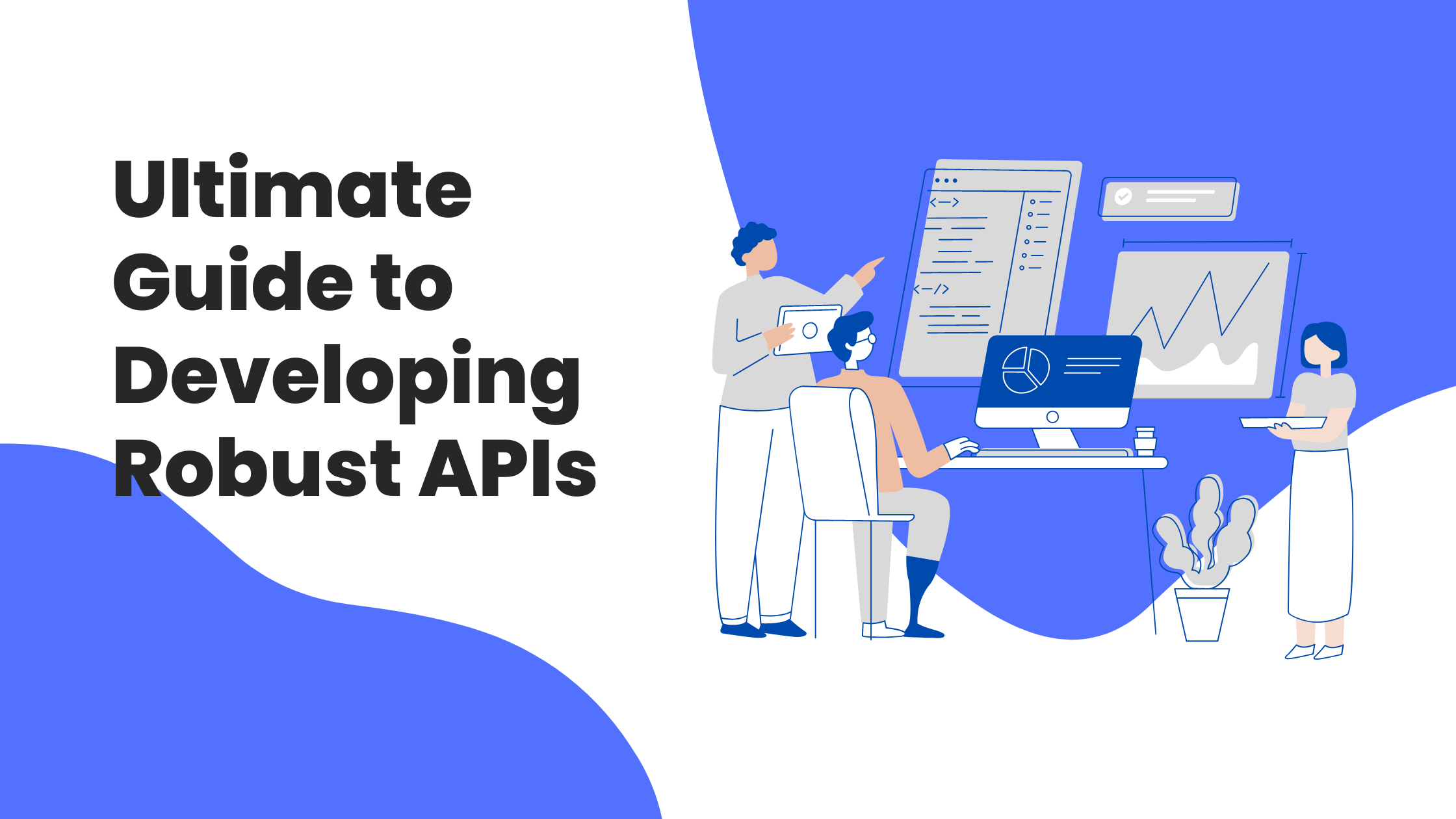
When developing applications, one of the critical things you ought to remember is that your application should have the capability of synergizing with other applications over a device to access their functionalities. This is what APIs are for.
API or Application Programming Interface can be thought of as the connectors between different pieces of code. They essentially allow for the development of applications that access data and features which are inbuilt into other applications and services. There may be times when you, as a developer, want to use different applications’ functionalities to create a more seamless experience for your application. APIs help you do exactly that.
With constant progress in the IT industry, there is also constant pressure to release more functionality at an exponential rate. As a cloud software provider, you can leverage APIs to leverage each other’s functionality to reduce the hassle.
The growth and adoption of APIs have sky-rocketed in the last decade. For example, Netflix can stream over 200 different devices thanks to its API, and 50% of Salesforce transactions are done through its API. For such huge returns, enterprises invest huge investments, and APIs have become a core building block in the IT industry over time.
In this article, we will see how you can build robust APIs and what ROIs you can expect.
Definitions
Before we dive into how we can start developing, as beginners let us understand what a few key terminologies.
- Latency is the measure of delay or the time an API takes to request and receive a response.
- REST API: It is a preferred style of API architecture. It allows for APIs to interoperate between different apps and devices over the internet.
- HTTPS Methods: HTTP methods tell your API what you want to do with it. There are four types of HTTPS methods, i.e. GET, POST, PATCH, and DELETE.
- Request and Response: Refers to different types of HTTPS methods listed above. They are pretty much what their names suggest. To utilize the API, you need to make calls to it. That may entail requesting the API to GET data, and the response would be what the API brings back.
- Headers are used to pass on additional information with the request and response.
- API Keys: These keys are primarily tools for authentication and verification of the user utilizing the API.
- JSON: JSON or JavaScript Object Notion can be considered a language with two applications used to communicate through the API. JSON is used for applications or servers and web applications to exchange data over the web.
- GET: It is an HTTPS method to request data from a server at a specified source.
- POST: It is used to create new information.
- PATCH: You can use this method to modify existing information.
- DELETE: As the name suggests, you can remove existing information using this method.
- Endpoint: Points where the interaction between the API and a server happens is known as Endpoints.
- OAuth: It is an authorization framework or open-standard authorization for APIs. Developers use it to provide open, secure, and limited access to the end-users data for usage of third-party applications or websites without giving them access to their passwords.
Web APIs
These are APIs that can be accessed using the HTTP protocol. They are primarily inclusive of APIs that are used to communicate with the browser and include services such as web notifications and web storage. There are different access and security levels with the various Web APIs categories. They can be classified into four broad categories.
- Open APIs
Open a.k.a. public APIs, are available to developers with minimal restrictions. These can require a quick registration with an API key or may be completely public. They are primarily intended for external users.
- Private APIs
Unlike Open APIs, they cannot be freely accessed by developers. They are primarily meant for internal teams to enhance productivity and utilization of services. Teams can utilize each other’s tools, data, and programs. This has various advantages over conventional integration techniques like a standard interface for connecting multiple services, security and access control, and an audit trail of system access.
- Partner APIs
Enterprises or developers can access partner APIs post an onboarding process and authentication, which the host institution does. They are primarily used for temporary access to proprietary tools or paid services.
- Composite APIs
Composite APIs have multiple endpoints, and they are a culmination of different services and data APIs. They can perform multiple tasks in a single call, making the process faster and reducing the server load.
Web API typically refers to both sides of computer systems communicating over a network, the API services offered by a server and the API given by the client, such as a web browser.
The server side of the web API, which is a programming interface to a defined request-response message system and is typically referred to as the Web Service.
Basic Architectures Used
There are various architectures for web services, out of which the following ones are the most popular.
- JSON-RPC and XML-RPC
JSON-RPC uses JSON for the encoding of the calls. RPC is a remote procedural call protocol, whereas XML-RPC uses the XML format for encoding calls. There are some key features which differentiate JSON-RPC from the other two in the list:
- Their primary use case is to call methods.
- The URI or Uniform Resource Identifiers can identify the server, but it does not contain any information within its parameters.
- REST
REpresentational State Transfer or REST is one of the most dominant API architectures preferred by developers. It is highly data-driven. and uses formats such as HTML, XML, JSON, and many more. A REST API adheres to some basic architectural constraints like:
- Client-server architecture
- Statelessness
- Cacheability
- Layered System
- SOAP
Simple object access protocol, or SOAP, is another preferred web API protocol. It has been developed to operate over various communication protocols, including HTTP, SMTP, TCP, and many more. It has specifications like:
- A process model
- Extensibility model
- Protocol binding rules
- Message construct
There is much literature that can highlight in detail what individual differences these styles of architecture have and which one would suit you for your project.
Tools for API Development
Below are five tools that are dominating the development space when it comes to APIs.
- JMeter: JMeter is an open-source API development tool that is often used for testing RESTful APIs.
- Swagger: One of the most popular API development platforms for RESTful APIs. It is also an open-source tool and pro version which has helped many developers around the globe to deliver and deploy amazing APIs.
- SoapUI: Another prominent API testing tool for web service testing. It can check both SOAP and REST web services. It is available to developers in both open-source and pro versions; naturally, the pro version facilitates additional functionalities.
- Apigee is an API development and management tool made available by Google to the masses. It is helpful if you are developing connected apps or, as an enterprise, updating legacy apps/facilitating data transfer between services and apps.
- PostMan: With PostMan, you can build and test your APIs. Open sources and plug-and-play have many features that allow you to perfect your APIs and make them faster than the other tools.
Again, you can choose from many other tools available on the net based on the convenience of your team and the project.
Essential Pointers for API Development
Now that we have the basics with us, let’s understand what developers should keep in mind to create robust APIs.
A wise man once said that APIs should be developed according to the context. You can either create APIs that can be chatty or chunky. A chatty API requires multiple calls to complete a common task, whereas a chunky API, which is quite the opposite, includes much more information and does much more in a single call.
As a developer, you must ask yourself many questions to perfect your work. Questions like what the user will want, which part of your API could become a bottleneck, which information should be grouped, and many more.
Apart from this, below are some pointers about features that every developer should keep in mind while developing APIs.
- Query, Filter, Sorting & Pagination
When you have been collecting data for a long time, eventually, you will start seeing a delay between a request for data retrieval and response for some resources.
To fix this, you can cache your objects/ and or create pagination/ filtering.
Once sorted, you can ensure that the users receive the data per the requirement, modification, and the specific condition applied. Pagination deals with things like how much data you should display and what would be the required frequency. These things can ensure minimum processing time, good response time, and very high levels of security.
- Authentication & Authorization
Ensuring that you have the right people hitting your endpoints is what authentication means. Also, ensuring that only the people who you want and who are verified can perform specific functions is termed as authorization. JWT, OAuth, and OAuth2 are some known specifications that can help you deal with this subject. But in some cases, you may also have to step outside the box and create new flavours of security tiers to meet specific requirements.
- Wrappers
In the tech world, a wrapper is an entity or framework that can be used to wrap or mask another item. It serves primarily two agendas. First and foremost, you can convert your data into a compatible format. Second, it can help you simplify complex entities or make a program perform complicated tasks easily using what is known as abstraction.
Using this concept, in API development, you can encapsulate multiple API calls into a single wrapper and reduce the complexity of using functions. Thus, developers do not need to depend on real-time interaction with the APIs and can automate many API-reliant processes.
- Cache
Want to retrieve your resource amazingly fast? Developing a cache strategy is vital. Once you figure out how to get the data already for consumption in an in-memory database, you will figure out how to reduce your costs. Tools like Memcached and Redis will be convenient for doing this.
- HATEOS
HATEOS stands for Hypermedia as the application state of the engine. It is an approach to allow clients to dynamically and autonomously interpret a resource’s current state and transitions that can be initiated arising from this state.
Using standardized RESTful services, which can be accomplished using HATEOS, allows your clients to use the resources efficiently. This is done as navigation possibilities between the states will be listed in every hit on your resources.
Conclusion
In this article, we have covered many points, including the basic definitions, tools, categories, and features that aim to guide you towards developing robust APIs. You must focus on creating an API that ensures a seamless handshake and is consistent, stable, flexible, and secure. If you keep these points in mind, then you should be good. But do keep searching and experimenting. The road to robust APIs is filled with much experimentation.